Go Practical! • The Applied Go Weekly Newsletter 2024-11-10
Your weekly source of Go news, tips, and projects
Go Practical!
Hi ,
Today's issue is all about practical Go! Build a system monitor, write secure code, understand sync.Once
, listen to the creators of Elvish and Fyne, take part in some aha moments, learn how to apply TeeReader
and MultiReader
to an audio mixing problem, or see a non-linear synthesizer in action.
I love this broad spectrum of use cases where Go can absolutely shine.
Featured articles
Build a System Monitor TUI (Terminal UI) in Go
Every tutorial has a purpose, but this one has two:
- Learn how to read system info
- Learn how to build a TUI with BubbleTea
Writing secure Go code | Jakub Jarosz
Don't put off securing your Go app because you think you cannot start without a dozen security specialists and pen testers at hand.
A number of rather straightforward steps and actions can already get you a long way.
(But having those security specialists around isn't exactly a bad option, either.)
Go sync.Once is Simple... Does It Really?
Executing a function once and skipping it on repeated calls seems a simple task, but concurrency makes things complicated under the hood, as always.
Podcast corner
go podcast() | 047: Fyne toolkit with Andy Williams
Fyne is a desktop app toolkit for Go, and the founder of Fyne.io and FyneLabs.com, Andy Williams, is the guest of this episode.
Writing a shell in Go
"Of all possible side projects, why a shell?" Qi Xiao, author of Elvish, explains Johnny why he decided to write a shell, and how development has been going on during the recent eleven(!) years.
Spotlight: Conventions in Go (a refresher)
Good conventions make reading, sharing, and talking about code much easier.
Recently, I posted a quick tip about using the Must
prefix to name a function that panics if receiving an illegal input. The reactions showed me that there is a great interest in learning and using conventions for writing code.
So here are a few Go conventions that are easy to stick with and have a great impact on readability. (I'll omit Must .)
In multi-value returns, put the error value last.
If you write a function that returns one or more values plus an error value, put the error value last in the list of return values:
func No(yes bool)(bool, err) {…}
Don't use naked returns.
If you name return arguments, you don't need to use them in the return directive. Do it anyway!
// bad
func naked()(first, middle, last, email string) {
…
return
}
// good
func proper()(first, middle, last, email string) {
…
return first, middle, last, email
}
Return early
Implement error handling as if err != nil
rather than if err == nil
. The former is much more common, and using the latter can trick a reader into thinking that the if
block handles an error whereas it is the "happy path".
So test for err != nil
and return inside this if
block if the error cannot be handled. Doing this,
- you minimize indenting, because if you used
err == nil
instead, every subsequent error check would add another level ofif
nesting - you keep the "happy path" to the left of the screen, for easy reading.
Package names
Name your packages so that the name relates to what the package proviedes. Avoid util
, helper
, common
like the plague.
Avoid stutter
If your package name is vectordb
, don't create functions like NewVectorDB
or OpenVectorDB
. Remember they will be used along with the package name: vectordb.NewVectorDB()
, vectordb.OpenVectorDB()
. Avoid this stutter! New
or Open
is perfectly fine.
Interfaces are Doers, and they are small
Interface names are kind of special in Go. Where other languages prepend a capital I to the name, Go chooses names ending in -er, such as Reader, Writer, or Closer.
Besides that, interfaces are also small—a single function per interface is quite common.
These two conventions ensure maximum composability.
Getters without Get
Methods that read a value should not be prepended with "Get". For example, if your method returns a URL, name it URL()
rather than GetURL()
.
Gofmt
Finally, the convention that is probably the one with the farthest and most visible impact is "Use gofmt
." Go's code formatter ends all debates about where to put an opening brace. As a Go proverb says, "Gofmt's style is no one's favorite, yet gofmt is everyone's favorite." So add Gofmt to your favorite IDE or editor if you haven't. Usually, you would add the Go Language Server gopls
that comes with gofmt
out of the box.
More
Conventions are weaker than hard rules; yet, following the various conventions in Go always pays out. You might argue that some (or all) of these conventions are "nothing but" styling rules. I would not disagree; there is a lot of overlap in the meaning of the words. In fact, you find many of the above conventions in the Google Go Style Guide, along with many other styling rules and recommendations. Worth a read!
Quote of the Week: A principle of learning
An underappreciated principle of learning is that you can't understand the solution to a problem until you have the problem.
More articles, videos, talks
Golang Aha! Moments: OOP
If you come to Go with years of object-oriented programming deeply engraved in your muscle memory, you will have a few deja-vu moments while reading this article.
A Golang Pipeline Abomination
A nice exercise in both how to iterate towards a solution and how to use io.TeeReader
and io.MultiReader
for mixing audio loops with ffmpeg
.
UPDATE: The original is gone, but archive.org has an archived version.
Live improv with Signls v0.1.2 - YouTube
Just watching the video of this sequencer generating music can be mesmerizing.
Projects
Libraries
GitHub - Djancyp/luna: go react ssr
Server-side rendering for React, for better SEO and improved response times. Based on Echo.
GitHub - jongyunha/shrinkmap: ShrinkableMap is a high-performance, generic, thread-safe map implementation for Go that automatically manages memory by shrinking its internal storage when items are deleted. It provides a solution to the common issue where Go's built-in maps don't release memory after deleting elements.
The author frequently encountered issues with code where long-lived maps don't shrink after deleting entries, and so they wrote ShrinkableMap
.
GitHub - orsinium-labs/jsony: ⚡️ A blazing fast and safe Go package for serializing JSON
If your app mostly serializes JSON (and rarely needs to deserialize JSON), this package may give your app a speed boost.
GitHub - idsulik/go-collections: Go package that provides implementations of common data structures including a double-ended queue (Deque), a linked list, a queue, a trie, a stack, a priority queue, a binary search tree, a graph, and a skip list.
Collections of data can vastly vary in internal structure and behavior. This "mega-package" provides a baker's dozen of data structures that cover a wide range of use cases.
GitHub - Kei-K23/spinix: Spinix 🌀 is a Go package that provides terminal-based highly customizable and performance loading animations, including spinners and progress bars.
Spinners with style!
Tools and applications
GitHub - 0x4f53/secretsnitch: A fast and continuous secret scanner in Go
Does your website involuntarily expose secrets? Secretsnitch helps you to find API keys, password variables, URLs with embedded authentication, ssh keys, .pem files, and other secrets that should not be accessible from the internet. Keep your website safe!
GitHub - tymbaca/wikigraph: Wikipedia articles graph parser
If you click the first link of any Wikipedia article, and repeat this on every subsequent article, you'll most probably reach the Philosophy page.
If you click all links in an article and repeat this, then... well, then you'll be quite busy for the next years. Better let this app generate a graph of all Wikipedia articles that can be reached from a given article.
notepad.moe
Another pastebin clone: nice. Another Go+HTMX+Postgres app for inspection: nicer!
GitHub - adelowo/httpreturnchecker
If you see a coding error happen repeatedly, it's time to write a linter. This is what the author of httpreturnchecker
did after observing too many missing returns after writing an HTTP response.
GitHub - idsulik/swama: Swama is a powerful command-line interface (CLI) tool for interacting with Swagger/OpenAPI definitions
Swama takes an existing Swagger/OpenAPI specifications and lets you view endpoint information or run a mock server.
GitHub - magooney-loon/milkshaker_visualizer: CLI mp3 player + visualizer
Staring at your computer screen and nothing happensß Turning the music on doesn't really help? Then try milkshaker_visualizer
, an mp3 player that turns your terminal into a discotheque.
GitHub - MrNemo64/go-n-i18n: A i18n code generation tool for type safe and feature rich internationalization
Internationalization (or i18n) the easy and maintainable way:
- Write a JSON file with string definitions and translations
- Generate code
GitHub - darwishdev/sqlseeder
sqlseeder
seeds databases from Excel sheets or JSON files, but it does more than just filling data table by table. It is possible to model relationships in the source data that sqlseeder
turns into proper table relations.
GitHub - sensepost/gowitness at dailydev
Take screenshots of web pages, save them to SQLite, and view them in a WebUI! Or save them to JSONLines or CSV (but then without having a WebUI).
Utilizes Chrome Headless for the tedious work.
GitHub - loxilb-io/loxilb: eBPF based cloud-native load-balancer for Kubernetes|Edge|Telco|IoT|XaaS.
LoxiLB, a load-balancer, has become a CNCF sandbox project. Congrats!
GitHub - 0xb-s/chat-app: A simple terminal chat application for real-time messaging.
Turns out that half of the /r/golang community seems to work on a chat app (ok, three peeps as I am writing this, plus OP).
Completely unrelated to Go
AI Data Center Boom and Renaissance of Sustainability Tech
I must admit, I have turned into a daily user of AI services. I query LLMs on documents and code, let them check my writing for typos and grammatical flaws, let them sometimes produce ideas or ask them to help me understand a particular topic.
As AI models become more and more powerful and widespread, however, their consumption of energy and resources goes through the roof. Aysu Kececi takes a close look at the state of affairs and ruthlessly reveals the mind-boggling numbers that AI is heading towards. But she also presents solutions that are already actively pursued, from harvesting sustainable energy big style to using AI itself to help reduce power consumption. With an intro (or foreword?) by Michael Spencer.
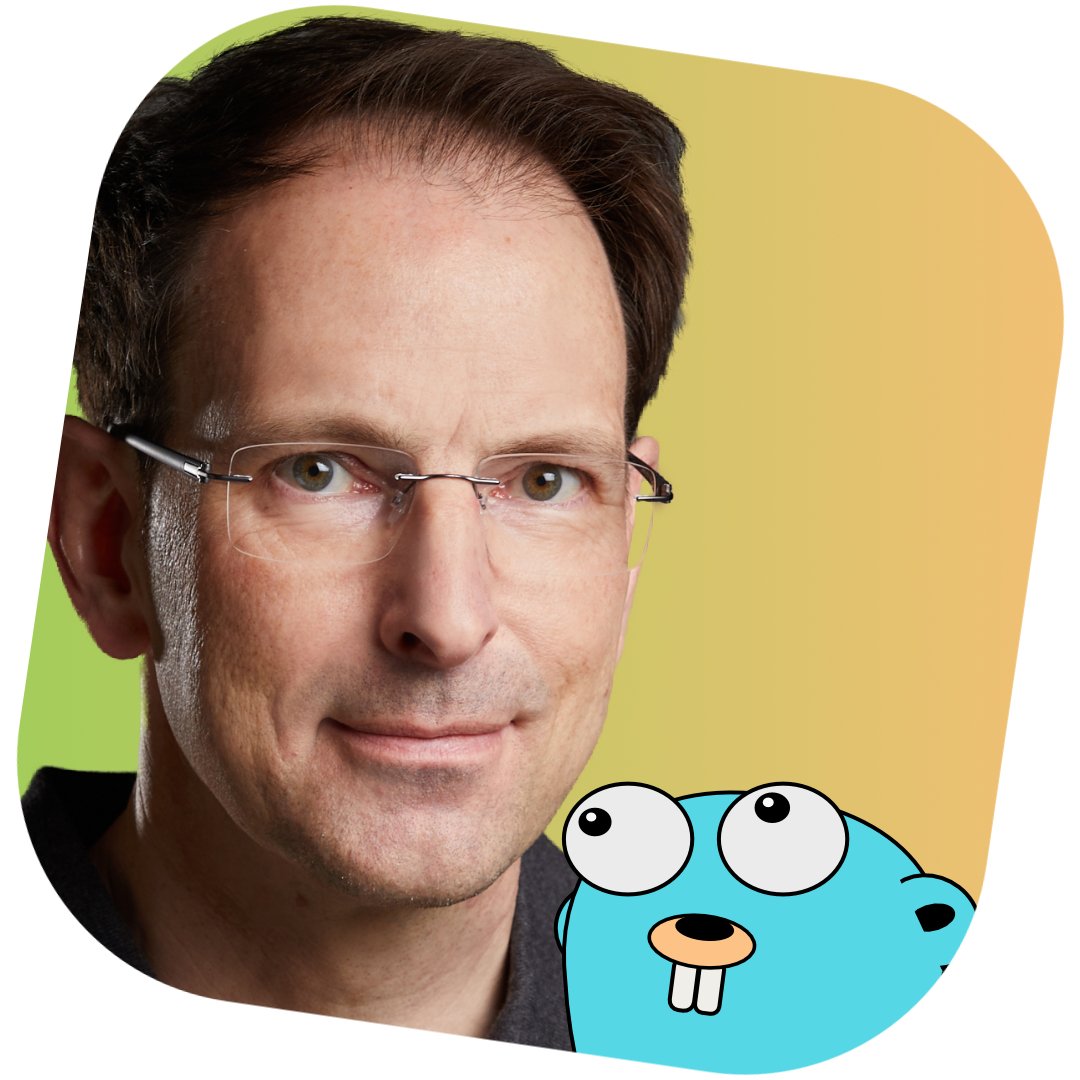
Happy coding! ʕ◔ϖ◔ʔ
Questions or feedback? Drop me a line. I'd love to hear from you.
Best from Munich, Christoph
Not a subscriber yet?
If you read this newsletter issue online, or if someone forwarded the newsletter to you, subscribe for regular updates to get every new issue earlier than the online version, and more reliable than an occasional forwarding.
Find the subscription form at the end of this page.
How I can help
If you're looking for more useful content around Go, here are some ways I can help you become a better Gopher (or a Gopher at all):
On AppliedGo.net, I blog about Go projects, algorithms and data structures in Go, and other fun stuff.
Or visit the AppliedGo.com blog and learn about language specifics, Go updates, and programming-related stuff.
My AppliedGo YouTube channel hosts quick tip and crash course videos that help you get more productive and creative with Go.
Enroll in my Go course for developers that stands out for its intense use of animated graphics for explaining abstract concepts in an intuitive way. Numerous short and concise lectures allow you to schedule your learning flow as you like.
Christoph Berger IT Products and Services
Dachauer Straße 29
Bergkirchen
Germany