Optimizations, Small And Big • The Applied Go Weekly Newsletter 2025-03-16
Your weekly source of Go news, tips, and projects
Optimizations, Small And Big
Hi ,
Slow apps are a nuisance and can cost real money. Hence, optimization should always be a regular part of the development workflow. In some cases, optimizations are spectacular, such as the rewrite of the TypeScript compiler in Go (see the Featured articles section). In other cases, the result may be subtle but still worth the effort (see the Spotlight section).
An app's architectural design, however, can decide upon the largest performance benefits (or losses). So be rigorous about designing your app for performance. That's not premature optimization. Fiddling with peephole optimization before knowing the whole performance picture is.
But eventually, your app may face a situation where a spectacular rewrite or a subtle punctual optimization are unavoidable. I wish you the sensibility to spot those situations and the determination to find and fix the performance bottlenecks.
– Christoph
Featured articles
A 10x Faster TypeScript - TypeScript
Go has a track record of speeding up legacy software—or, at least, it plays a significant role in success stories of this kind.
Now Microsoft chose Go for porting the TypeScript compiler and tools, and the results are impressive: most build times are 10 times faster, at a substantially reduced memory usage.
In this article, Anders Hejlsberg, lead architect of TypeScript (and original designer of C#, Delphi, and Turbo Pascal) explains the goals and current status of the project.
Not surprisingly, the announcement has stirred up fans of other languages who felt that their favorite language hasn't been taken closely enough into consideration. Microsoft reacted to these concerns by explaining the reasons in the project's FAQ.
The main point is that "Idiomatic Go strongly resembles the existing coding patterns of the TypeScript codebase, which makes this porting effort much more tractable."
Then, and this may come as a surprise to some, Microsoft considers Go's garbage collector a big positive factor. "Go also offers excellent control of memory layout and allocation (both on an object and field level) without requiring that the entire codebase continually concern itself with memory management. "
Furthermore, "We also have an unusually large amount of graph processing, specifically traversing trees in both upward and downward walks involving polymorphic nodes. Go does an excellent job of making this ergonomic, especially in the context of needing to resemble the JavaScript version of the code."
Building a Secure Session Manager in Go
Building a session manager is easy in Go, but is the result secure? Mohamed Said shows how to build a session manager with strong session IDs, secure session cookies, proper session expiration and (in a follow-up article linked at the end) protection against cross-site request forgery (CSRF).
Is sqlc the BEST Golang package to work with SQL? - YouTube
Is database/sql
, sqlx
, sqlc
, or an ORM the best choice for working with a SQL database? Alex Pilutau explores all three approaches, ultimately advocating for sqlc
as a middle-ground solution that reduces boilerplate code while maintaining type safety and control over SQL queries.
Podcast corner
Cup o' Go: ✍️ Rewriting all the things in Go! 🎉
Microsoft ported the TypeScript compiler to Go. Jonathan and Shay take this one step further: Why not port everything to Go?
Fallthrough: An Exploration of APIs, Versioning, & HTTP
Web APIs want to be designed, documented, and versioned well. Jamie Tanna and the panel discuss this and more in this Fallthrough episode.
go podcast() 054: Datastar with Delaney Gillilan
This episode's guest is Delaney Gillian, author of Datastar, a web app framework combining HTMLX and Alpine.js.
Spotlight: Optimize memory usage with slices.Clip
Problem: Unused slice capacity wastes memory
Slices in Go are dynamic arrays with a length and capacity. When you shorten a slice using [:n]
, the underlying array retains its capacity. This may be useful if the shortened slice will grow to about the array's size again, but if the short slice will remain short or is unlikely to grow back to the old size (and the context allows to know this at compile time), you might want to return the unused memory back to the operating system. (Talking about long-running apps here.)
Solution: Use slices.Clip
to reclaim unused capacity and reduce memory overhead.
How It Works
When you create a large slice and then resize it (e.g., by slicing it to a smaller size), the original backing array still holds onto the allocated memory. slices.Clip
trims the slice’s capacity to match its length. The runtime then can get rid of the unused memory.
In a nutshell:
large := make([]int, 1_000_000) // 1M elements
clipped := slices.Clip(large[:10]) // Trim capacity
Some heap stats printing (see the full code in the Playground confirms the effectiveness:
Baseline mem : HeapInuse = 0.41 MB
clipped's capacity: 10
After clipping: HeapInuse = 8.03 MB
After cleanup : HeapInuse = 0.40 MB)
So if you have a long-running app that generates possibly long-lived large slices that might not grow to their previous size after reuse, consider clipping the slice.
I hear you asking: what about simply creating a new slice and copying the content over, like so:
oldSlice := largeSlice[:10]
newSlice := make([]int, len(oldSlice))
copy(newSlice, oldSlice)
Well, the slices.Clip
variant is -
- Cleaner: One line versus three lines of boilerplate
- Clearer: Clearly communicates to other developers what you're trying to accomplish
- Optimized-er (ouch): The compiler can potentially optimize
slices.Clip()
better than manual allocation plus copying
Note that the third aspect depends a lot on the circumstances and needs to be verified through benchmarks for every given case. Don't expect too much performance improvement, though (if any). The main advantage of slices.Clip()
over manual allocation is clarity of intent.
Quote of the Week: A grocery list of gripes
I have a grocery list of gripes with Go... and it is by far my favorite language to write in.
More articles, videos, talks
Running your Go tests in Github Continuous Integration
Dolthub plans to beta-release Doltgres, the Postgres version of Dolt, in about a month. (For me, that's already the main message!) This means running tests always and everywhere, including the CI pipeline. In this article, Zach Musgrave explains how DoltHub uses GitHub Actions to run Go tests, particularly if the tests need a long-running binary like Postgres.
Archiving the dolthub/swiss GitHub Repository
Heads up, dolthub/swiss
users: The Dolt team archives this repository in favor of the "multiple compelling swiss table implementations in Golang available within the ecosystem".
Goroutines in Go: A Practical Guide to Concurrency
Goroutines for newcomers, by Deven J.
Two mul or not two mul: how I found a 20% improvement in ed25519 in golang | Storj Engineering Blog
How Egon Elbre made the Edwards 21559 algorithm up to 20% faster—coming to you in Go 1.25.
Benchmarking: What You Can't Miss in Go 1.24 | Jakub Jarosz
The new b.Loop()
operator is a simple innovation with a big impact to writing benchmarks. Jakub Jarosz digs into the details.
Nil comparisons and Go interface
nil
s can be confusing if they hide in data types. Redowan Delowar summarized what he learned from Russ Cox about nil
and interfaces.
Projects
Libraries
GitHub - vfslite/vfslite: VFSLite is a lightweight virtual file system. Open-source and self-contained in a single file.
A virtual file system for learning purposes.
Lets build a Database from scratch
A SQL database built in plain Go, with no external dependencies? Venkat did it, mostly to learn how things work under the hood.
GitHub - GianlucaP106/gotmux: Go library for tmux
Manage tmux
sessions, windows, and panes from your Go app
Tools and applications
GitHub - surbytes/gitusr: A fast and simple CLI tool built in Go that allows you to effortlessly switch between multiple global Git users.
You can define Git users on a per-repo basis, but what if you need to switch users independently of the current repo or working directory? gitusr
fills this gap. Switch the current global git user within seconds.
GitHub - thevxn/xilt: A utility for parsing Common and Combined Log Format (CLF) log files and storing them in SQLite for further analysis. Built with concurrency in mind.
Need to do a little analysis of CLF-formatted log files but without reaching out for heavy-weight tools? xilt
could be an alternative.
GitHub - ddoemonn/go-dot-dot: A PostgreSQL database explorer TUI (Terminal User Interface) application written in Go.
Browse PostgreSQL databases in your terminal!
Completely unrelated to Go
Native Git support in Zed
Zed, the resource-friendly code editor for macOS and Linux, now comes with an integrated Git UI. I followed the development for a few days via the developer preview version and am happy to see it released to the stable version. While a couple of features are still under development (such as a visual Git history), the UI is already usable and shines with a clear UX.
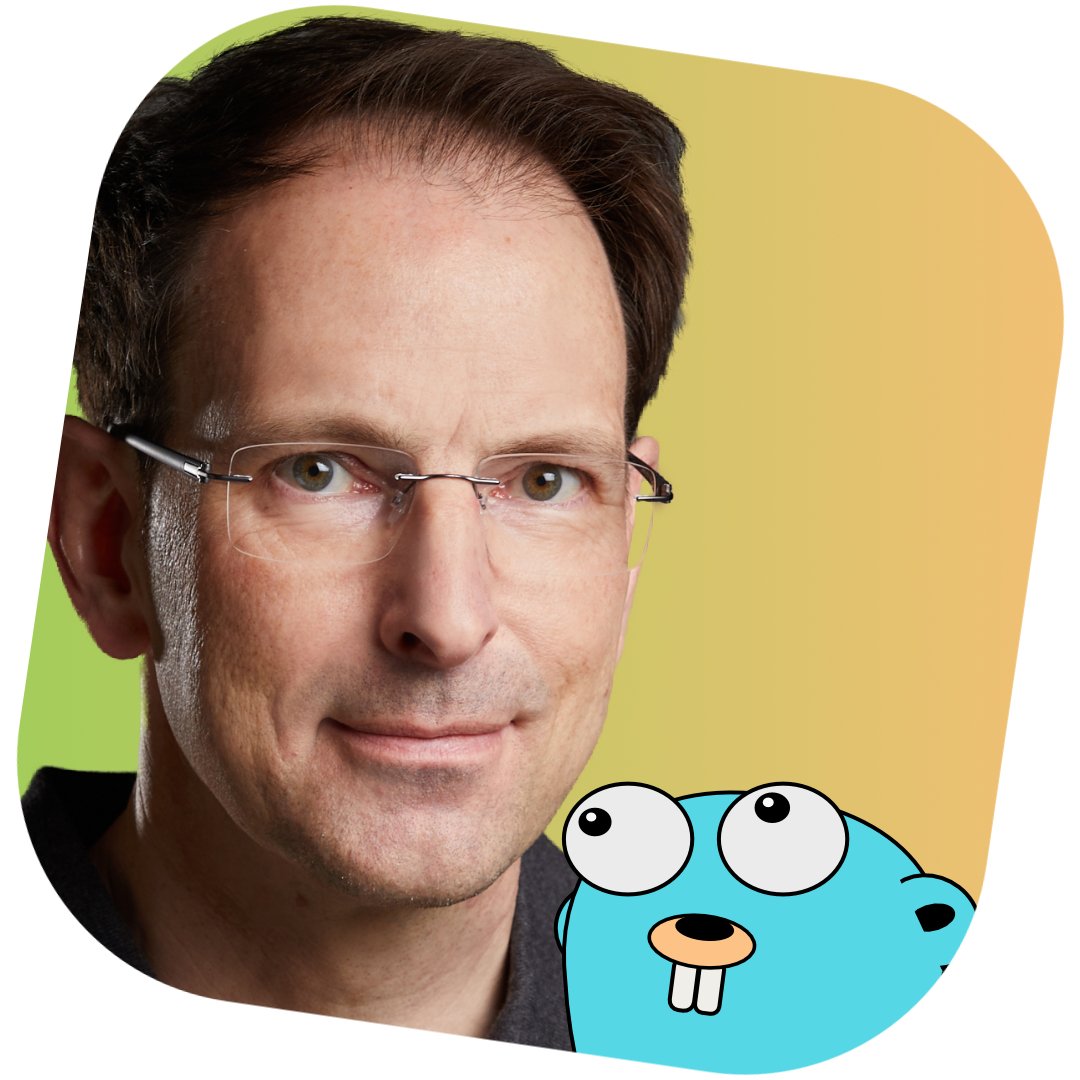
Happy coding! ʕ◔ϖ◔ʔ
Questions or feedback? Drop me a line. I'd love to hear from you.
Best from Munich, Christoph
Not a subscriber yet?
If you read this newsletter issue online, or if someone forwarded the newsletter to you, subscribe for regular updates to get every new issue earlier than the online version, and more reliable than an occasional forwarding.
Find the subscription form at the end of this page.
How I can help
If you're looking for more useful content around Go, here are some ways I can help you become a better Gopher (or a Gopher at all):
On AppliedGo.net, I blog about Go projects, algorithms and data structures in Go, and other fun stuff.
Or visit the AppliedGo.com blog and learn about language specifics, Go updates, and programming-related stuff.
My AppliedGo YouTube channel hosts quick tip and crash course videos that help you get more productive and creative with Go.
Enroll in my Go course for developers that stands out for its intense use of animated graphics for explaining abstract concepts in an intuitive way. Numerous short and concise lectures allow you to schedule your learning flow as you like.
Christoph Berger IT Products and Services
Dachauer Straße 29
Bergkirchen
Germany