Burrito time: Wrap that error? • The Applied Go Weekly Newsletter 2024-05-26
Your weekly source of Go news, tips, and projects
Do you remember the Go Tip from two weeks ago? I listed three anti-patterns, including dot imports. Dot imports are rightly said to pollute the namespace of the importing package and make understanding the code harder. But still, if certain imported identifiers are used intensely in the importing code, using them without the package prefix can be a legitimate wish. Today's Go Tip shows how to use imported identifiers without their package prefix and without obscuring their origin.
But first, don't miss these articles of the week:
Go Error Propagation and API Contracts
if err != nil { return err }
is said to be an antipattern. The opposite — that is, to always wrap an error — isn't always appropriate either. Matt T. Proud suggests looking at domain borders to determine whether to wrap an error.
Go Error Propagation and API Contracts
Go's old $GOPATH story for development and dependencies
"Before Go modules, Go had only one single workspace." Or had it? Chris Siebenmann considers the old GOPATH to be a multi-workspace concept similar to Python's virtual environments.
Go's old $GOPATH story for development and dependencies
Nailing the Technical Interview
Why a Least Recently Used cache is a great example of a useful interview challenge. With code snippets in Go.
Nailing the Technical Interview | David Campbell | Medium
2024 Stack Overflow Developer Survey
Raise your voice by participating in the SO Developer Survey. The gates are open until June 7th.
2024 Stack Overflow Developer Survey
Podcast corner
Cup o' Go: 🕸️ With great power comes great responsibility, or why not to use the linkname directive
In this weeks episode, Miki Tebeka, author of Effective Go Recipes, joins Jonathan Hall to discuss proposals, conference updates, and more stuff that happened this week.
go podcast(): 038: Finally, found a good use case for Go's plugin
Honestly, I thought that Go's plugin package is on its way out, but Dominic St-Pierre found a compelling, albeit special, use case.
Go Time: Migrating from PHP to Go
So you are a PHP developer who moves over to Go? That's like migrating to a totally different planet! Don't panic, you're not alone! Matt Boyle & Chris Shepherd share their PHP-to-Go stories in this Go Time episode.
Go tip of the week: No dot import and no package prefix
Dot imports are frowned upon, and rightly so.
If you import a package through a dot import, all the package's exported identifiers can be referred to directly, without the need for prefixing them with their package name. Effectively, these identifiers appear to belong to the current package, making the code harder to understand. Hence, dot imports should be avoided.
In the README of package BooleanCat/option
, I found an interesting alternative that can be considered if the number of "aliased" identifiers is small. Why do I put "aliased" in quotes? Look at the following code, specifically at the variable declaration:
import (
"fmt"
"github.com/BooleanCat/option"
)
var (
Some = option.Some
None = option.None
)
func main() {
two := Some(2)
fmt.Println(two)
}
So the "aliasing" is in fact a variable holding a function. This trick allows selectively "dot-importing" a few identifiers while keeping the rest behind the package prefix. This can be particularly useful for packages with a large number of exported identifiers of which only two or three are used frequently.
"But, Christoph, the option
package doesn't export much more than these two functions! The only other top-level identifier is Option
that package won't use directly (but only through Some
's and None
's return values), and the rest are methods."
This is true, but even then, the above technique is so much better because it declares Some
and None
in the same file as they are used. Imagine a dot import of the two. Unprepared readers would scan the file up and down, hoping to see where they are declared. (At best, the editor is able to reveal the nature and origin of Some
and None
through showing a popup driven by gopls
, the Go language server, but it's foolish to assume that every programmer and their dog uses sophisticated code editors. Post code with a dot import on a plain web page with no language server support and readers will be left puzzled.)
So: Instead of dot-importing, use local variables as aliases for imported identifiers, if you absolutely want to use them without their package prefix.
Quote of the week: Absurdity
Sometimes I want to laugh at the absurdity of foreign vocabulary like das Faultier (literally: „lazy animal“), but then I think of the English equivalent: sloth.
More articles, videos, talks
Boost performance of Go applications with profile guided optimization - YouTube
Profile-Guided Optimization (PGO) explained by Cameron Balahan, product lead for Go, and his colleagues Michael Pratt and James Ma.
Why didn't Go get a breakthrough in bioinformatics (yet)? | Bionics IT
Yes, why? Is Go too verbose? Or is it maybe that other languages were there first?
So we built a Reverse Tunnel in Go over HTTP/3 and QUIC | Flipt Blog
Or: "How we over-engineered a solution using some bleeding-edge tech"
The GoLand 2024.2 Early Access Program Has Started! | The GoLand Blog
JetBrains runs an early access program for new releases of the GoLand IDE, which is a great occasion for testing GoLand for free.
Implementing MVCC and major SQL transaction isolation levels | notes.eatonphil.com
Here is some advanced stuff by Phil Eaton for database lovers: "In this post we'll build a database in 400 lines of code with basic support for five standard SQL transaction levels: Read Uncommitted, Read Committed, Repeatable Read, Snapshot Isolation and Serializable." — and the article might actually contain all 400 lines of code.
Even if you don't plan to re-implement the database, the article contains some learnings about database transactions.
A JavaScript developer tries Go for the first time
I find it always interesting to learn how newcomers with various development backgrounds perceive Go. Bahaa Zidan is a TypeScript programmer, and he found quite a few things to love in Go. (Bonus points for not lamenting about error handling ;-)
Continuous Benchmarks in GO. How to efficiently integrate benchmarks… | by pulkit kathuria | web-developer | May, 2024 | Medium
Are you worried about the performance of your code? (If you develop high-traffic or time-critical apps, you should be.) Pulkit Kathuria shows you how to integrate benchmarking in your CI pipeline (using a GitHub Action workflow as an example).
Parsing Recursive Polymorphic JSON in Go · Shallow Brook Software
The world of data exchange could be so simple and easy if the devil didn't invent recursive polymorphic JSON. Here is how to fight that demon.
Announcing the stable release of the Azure Cosmos DB client library for Go - Azure Cosmos DB Blog
Azure Cosmos DB for NoSQL got a Go package.
Stack in Golang - TheDeveloperCafe
From a simple, slice-based stack of int
s to a generic, concurrency-safe linked-list implementation.
Why I don't use a third-party assertion library in Go unit tests
A third-party testing framework is another dependency outside your control. If plain Go tests feel too verbose, use a simple, generic Equal(), says Yawar Amin.
Projects
Libraries
GitHub - BooleanCat/option: Support user-friendly, type-safe optionals in Go.
Option types are a language feature that seems to rank high on the Go community's wish lists. Here is a type-safe option package.
GitHub - 0x3alex/gee: Evalute math and logical string expressions
Evaluate simple mathematical (no precedence rules at the moment) and logical expressions.
GitHub - swdee/go-rknnlite: CGO bindings to RKNN-Toolkit2 to perform Inferencing in Go on Rockchip NPU
Run AI inference models at home! (Requires some hardware like a Radxa Rock 5C (Lite).)
GitHub - orsinium-labs/tinymath: 📐 The fastest and smallest Go math library for constrained environments, like microcontrollers or WebAssembly.
Do math in tiny places!
GitHub - go-dew/dew: A lightweight, pragmatic command bus library for Go, designed to enhance developer experience and productivity.
No, if you only want to spawn a goroutine, you don't need dew
. dew
was created to serve as a unified interface between the presentation layer and the use case layer, to help create a simpler backend architecture.
GitHub - roblillack/spot: React-like desktop GUI toolkit for Go
An interesting new GUI library is in the making! Spot is built on top of FLTK for Linux and Windows, and Cocoa for Mac. (Native Windows controls are on the roadmap.)
Tools and applications
GitHub - adrianlarion/tsls: Displays summaryy about number of file types and their size
This CLI tool displays file statistics grouped by extension ("termination" in tsls
terminology). Want to know the number and the cumulated size of all JPEGs in a directory? Run tsls
.
GitHub - f01c33/b-mouse: Control your cursor with your keyboard with binary search
Move your mouse cursor with the cursor keys, wasd, or hjkl keys. Each step moves the mouse half the distance the previous one did. Eventually you'll arrive at the point you want to click.
GitHub - fredrikaverpil/neotest-golang: Reliable Neotest adapter for Go.
Neotest is a framework for integrating testing with NeoVim, and neotest-golang
is a new Go adapter. The author had some issues with the existing neotest-go
adpater (that seem difficult to resolve in the neotest-go
codebase) and decided to build a new one as a PoC.
GitHub - obarker94/go-observability-example: A brief working example of adding distributed tracing to a Go application and configuring OTEL, Tempo and more.
Learn about using OpenTelemetry in Go by inspecting a live example that runs with a single docker compose up
. Observe three Go servers with Loki, Tempo, and Prometheus (inside Grafana).
GitHub - alexisvisco/amigo: A database migration tool in Go language inspired by active record migrations.
Another DB migration tool? What's the USP? Author: "Here is an attempt to be as happy as I was with active records. It offers a powerful API to manage schema changes and supports auto-reverse migrations."
GitHub - ddddddO/packemon: Packet monster (っ‘-’)╮=͟͟͞͞◒ ヽ( '-'ヽ) TUI tool and Go library for sending packets of arbitrary input and monitoring packets on any network interfaces (default: eth0).
Network packet monitoring so low-level you hear the wires humming.
GitHub - maxpoletaev/goxgl: My little software 3D renderer
Who needs OpenGL or DirectX? Let Go do the hard work!
This 3D renderer has no dependencies other than the C library raylib.
GitHub - adrianlarion/affirmtempl-open: An affirmations web app. Made with Go, Echo, Templ, jQuery, materialize and more
Need some positive affirmations? Try easyaffirm.com or, if you don't want to leave traces on other people's servers, run the server locally.
GitHub - saalikmubeen/go-grpc-implementation: Golang + Gin + Docker + gRPC + NGINX
A gRPC project that showcases an HTTP server, an gRPC server, and an HTTP-gRPC gateway.
GitHub - f01c33/rx: Regex eXplorer, allows you to test your regexes with live-reload
There are regex builders/validators on the Web, bug firing up a TUI app is usually much faster. Type a test string and then build your regular expression. The result pane highlights matches and prints errors.
GitHub - vyshnav-vinod/pathfinder: A command line tool to move between directories easily. Written in Go
cd
to a directory without typing out the path.
GitHub - adhd42/oxigen: Social media image generator
Enter text, website URL, an image URL, a logo URL, and other stuff, et voilà! a social media image!
Completely unrelated to Go
Solving the Database Debate
Which database to choose? Preslav Rachev has a surprisingly convincing solution. I'd second this solution any time.
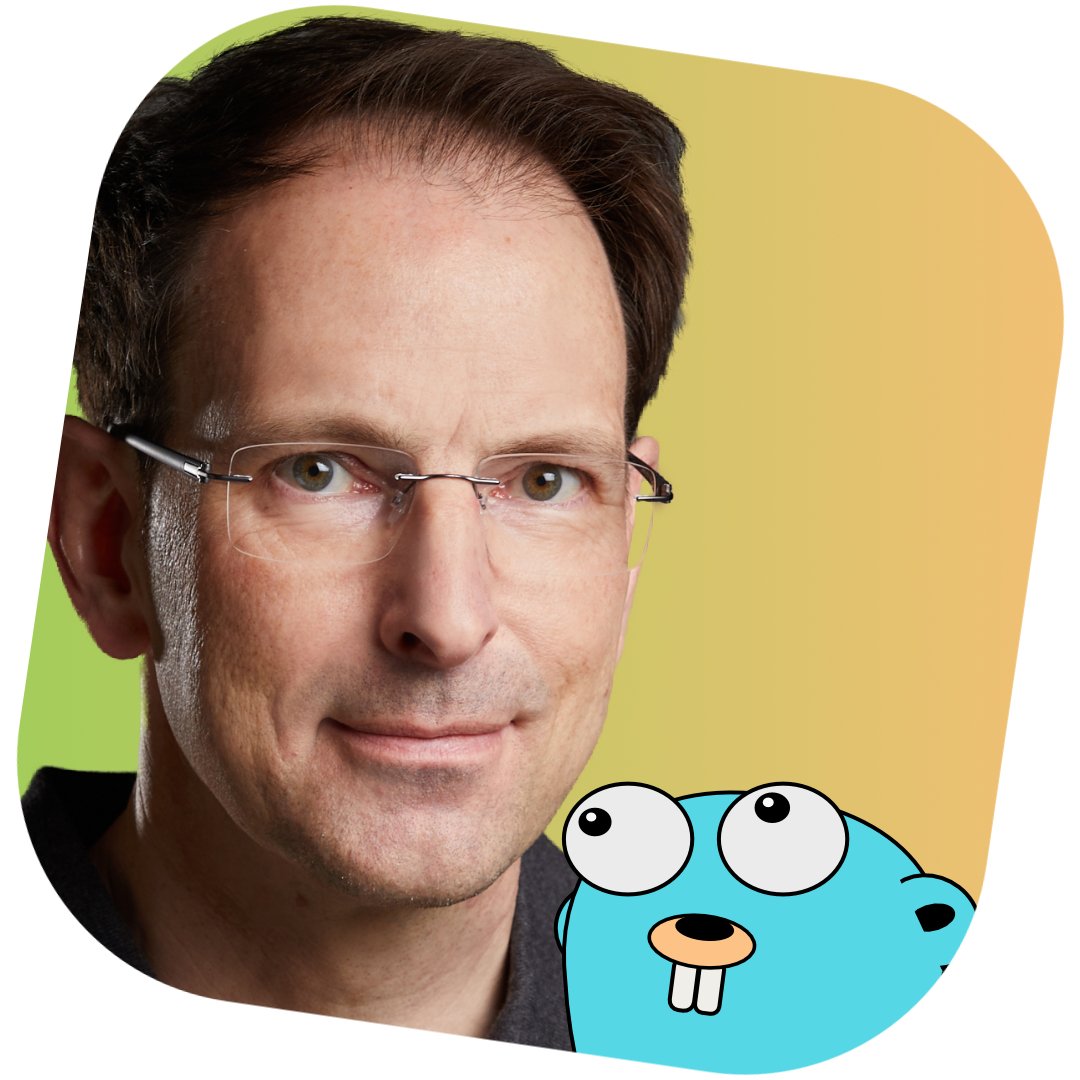
Happy coding! ʕ◔ϖ◔ʔ
Questions or feedback? Drop me a line. I'd love to hear from you.
Best from Munich, Christoph
Not a subscriber yet?
If you read this newsletter issue online, or if someone forwarded the newsletter to you, subscribe for regular updates to get every new issue earlier than the online version, and more reliable than an occasional forwarding.
Find the subscription form at the end of this page.
How I can help
If you're looking for more useful content around Go, here are some ways I can help you become a better Gopher (or a Gopher at all):
On AppliedGo.net, I blog about Go projects, algorithms and data structures in Go, and other fun stuff.
Or visit the AppliedGo.com blog and learn about language specifics, Go updates, and programming-related stuff.
My AppliedGo YouTube channel hosts quick tip and crash course videos that help you get more productive and creative with Go.
Enroll in my Go course for developers that stands out for its intense use of animated graphics for explaining abstract concepts in an intuitive way. Numerous short and concise lectures allow you to schedule your learning flow as you like.
Christoph Berger IT Products and Services
Dachauer Straße 29
Bergkirchen
Germany